MyBatis-Plus笔记
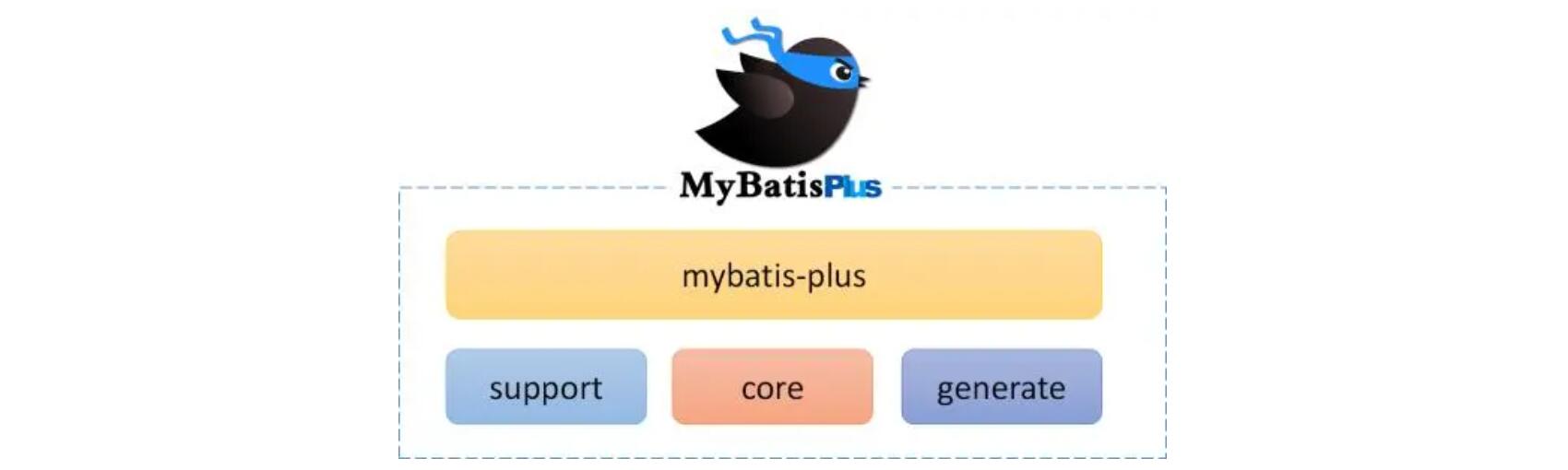
MyBatis-Plus 通用BaseMapper使用介绍
详细见快速开始
- 拥有 Java 开发环境以及相应 IDE
- 熟悉 Spring Boot
- 熟悉 Maven
现有一张 User
表,其表结构如下:
id | name | age | |
---|---|---|---|
1 | Jone | 18 | test1@baomidou.com |
2 | Jack | 20 | test2@baomidou.com |
3 | Tom | 28 | test3@baomidou.com |
4 | Sandy | 21 | test4@baomidou.com |
5 | Billie | 24 | test5@baomidou.com |
其对应的数据库 Schema 脚本如下:
|
|
其对应的数据库 Data 脚本如下:
|
|
::: danger Question 如果从零开始用 MyBatis-Plus 来实现该表的增删改查我们需要做什么呢? :::
初始化工程
创建一个空的 Spring Boot 工程(工程将以 H2 作为默认数据库进行演示)
::: tip 可以使用 Spring Initializer 快速初始化一个 Spring Boot 工程 :::
添加依赖
引入 Spring Boot Starter 父工程:
|
|
引入 spring-boot-starter
、spring-boot-starter-test
、mybatis-plus-boot-starter
、h2
依赖:
|
|
配置
在 application.yml
配置文件中添加 H2 数据库的相关配置:
|
|
在 Spring Boot 启动类中添加 @MapperScan
注解,扫描 Mapper 文件夹:
|
|
编码
编写实体类 User.java
(此处使用了 Lombok 简化代码)
|
|
编写Mapper类 UserMapper.java
|
|
开始使用
添加测试类,进行功能测试:
|
|
::: tip UserMapper 中的 selectList()
方法的参数为 MP 内置的条件封装器 Wrapper
,所以不填写就是无任何条件 :::
控制台输出:
|
|
::: tip 完整的代码示例请移步:Spring Boot 快速启动示例 | Spring MVC 快速启动示例 :::
小结
通过以上几个简单的步骤,我们就实现了 User 表的 CRUD 功能,甚至连 XML 文件都不用编写!
从以上步骤中,我们可以看到集成MyBatis-Plus
非常的简单,只需要引入 starter 工程,并配置 mapper 扫描路径即可。
但 MyBatis-Plus 的强大远不止这些功能,想要详细了解 MyBatis-Plus 的强大功能,请继续学习
MyBatis-Plus 通用IService使用介绍
一、引言
MP除了通用的Mapper还有通用的Servcie层,这也减少了相对应的代码工作量,把通用的接口提取到公共。其实按照MP的这种思想,可以自己也实现一些通用的Controller。
由于官网文档没有IService的使用教程,快速开始仅仅有Mapper的教程,故写了本文
二、IService使用
Service
Service层需要继承IService,当然实现层也要继承对应的实现类。
|
|
这里基本的增删改查就不一一演示了,演示几个特殊一点的方法。
getOne(),这个是方法返回结果不止一条则会抛出异常,如果想默认取第一条结果,可以给这方法传第二个参数为false。
|
|
saveOrUpdateBatch()
,批量新增或者修改方法,判断ID是否存在,如果ID不存在执行新增,如果ID存在先执行查询语句,查询结果为空新增,否则修改。
|
|
接下来说一下基于lambda的相关操作
|
|
Maybatis-Plus lambdaQuery和mapper中EQ、NE、GT、LT、GE、LE的对时间的用法及详解
1.等于当前时间
|
|
2.不等于当前时间
|
|
3.大于当前时间
|
|
4.小于当前时间
|
|
5.大于等于当前时间
|
|
6.小于等于当前时间
|
|
7.2个时间段是否相交
|
|
MyBatis-Plus 更新操作示例
|
|
MyBatis-Plus 分页查询示例
IPage封装了各种分页的信息,包括不限于总页数,当前页数,总个数,是否有上一页或下一页等
|
|
MyBatis-Plus 模糊查询及QueryWrapper<>常用条件方法介绍
|
|
我们直接创建测试类进行展示
|
|
在
QueryWrapper<>
中,有很多已经编译好的方法,我们可以直接进行调用,
|
|
在test4的方法中,使用likeRight进行查询带有K的数据
或者使用自己注入的sql语句,
|
|
当我们需要在Controller中使用方法时,需要把 QueryWrapper wrapper = new QueryWrapper<>();语句写入到Service层中
Service
这样我们在Controller中可以避免直接调用mapper层